Gerencie os seus certificados de SSL e verifique as expirações através de um simples e inteligente script, evitando assim que os navegadores impeçam o acesso ao seu site.
Por Adriano Valério
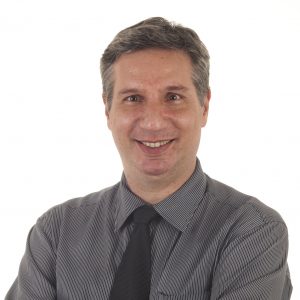
Primeiramente, temos que lembrar que a grande de maioria dos certificados de SSL (HTTPs) tem validade de 1 (um) ano, ocorre que muitas vezes a área de tecnologia não se atenta ao vencimento e o certificado de SSL expira. Isso acontece o tempo todo, na maioria das empresas.
Como verificar a expiração do certificado de SSL
USO: SSLexpiryPredictions.sh – [cdewh]
DESCRIÇÃO: Este script prevê os certificados SSL expirados com base na data final.
Opções
-c define o valor para o arquivo de configuração que possui detalhes server: port ou host: port.
-d define o valor do diretório que contém os arquivos de certificado no formato crt ou pem.
-e define o valor da extensão do certificado, por exemplo, crt, pem, cert. Por padrão o script utiliza a extensão .crt [a ser usado com -d, se a extensão do arquivo de certificado for diferente de .crt]
-w define o valor para gravar a saída do script em um arquivo.
-h imprime essa ajuda e sai.
Exemplos
Para criar um arquivo com uma lista de todos os servidores e seus números de porta para fazer um handshake SSL, use:
cat> servers.list
server1: porta1
server2: porta2
server3: porta3
(ctrl + d)
$ ./SSLexpiryPredictions.sh -c server.list
Execute o script fornecendo o local e a extensão do certificado (caso não seja .crt):
./SSLexpiryPredictions.sh -d /path/to/certificates/dir -e pem
Abaixo, está disponível o código fonte do script.
SCRIPT
#!/bin/bash
##############################################
#
# PURPOSE: The script to predict expiring SSL certificates.
#
# AUTHOR: ‘Abhishek.Tamrakar’
#
# VERSION: 0.0.1
#
# COMPANY: Self
#
# EMAIL: abhishek.tamrakar08@gmail.com
#
# GENERATED: on 2018-05-20
#
# LICENSE: Copyright (C) 2018 Abhishek Tamrakar
#
# Licensed under the Apache License, Version 2.0 (the “License”);
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an “AS IS” BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
##############################################
#your Variables go here
script=${0##/}
exitcode=”
WRITEFILE=0
CONFIG=0
DIR=0
# functions here
usage()
{
cat <<EOF
USAGE: $script -[cdewh]”
DESCRIPTION: This script predicts the expiring SSL certificates based on the end date.
OPTIONS
-c| sets the value for configuration file which has server:port or host:port details.
-d| sets the value of directory containing the certificate files in crt or pem format.
-e| sets the value of certificate extention, e.g crt, pem, cert.
crt: default
-w| sets the value for writing the script output to a file.
-h| prints this help and exit.
EOF
exit 1
}
# print info messages
info()
{
printf ‘\n%s: %6s\n’ “INFO” “$@”
}
# print error messages
error()
{
printf ‘\n%s: %6s\n’ “ERROR” “$@”
exit 1
}
# print warning messages
warn()
{
printf ‘\n%s: %6s\n’ “WARN” “$@”
}
# get expiry for the certificates
getExpiry()
{
local expdate=$1
local certname=$2
today=$(date +%s)
timetoexpire=$(( ($expdate – $today)/(60*60*24) ))
expcerts=( ${expcerts[@]} “${certname}:$timetoexpire” )
}
# print all expiry that was found, typically if there is any.
printExpiry()
{
local args=$#
i=0
if [[ $args -ne 0 ]]; then
#statements
printf ‘%s\n’ “———————————————”
printf ‘%s\n’ “List of expiring SSL certificates”
printf ‘%s\n’ “———————————————”
printf ‘%s\n’ “$@” | \
sort -t’:’ -g -k2 | \
column -s: -t | \
awk ‘{printf “%d.\t%s\n”, NR, $0}’
printf ‘%s\n’ “———————————————”
fi
}
# calculate the end date for the certificates first, finally to compare and predict when they are going to expire.
calcEndDate()
{
sslcmd=$(which openssl)
if [[ x$sslcmd = x ]]; then
#statements
error “$sslcmd command not found!”
fi
# when cert dir is given
if [[ $DIR -eq 1 ]]; then
#statements
checkcertexists=$(ls -A $TARGETDIR| egrep “*.$EXT$”)
if [[ -z ${checkcertexists} ]]; then
#statements
error “no certificate files at $TARGETDIR with extention $EXT”
fi
for file in $TARGETDIR/*.${EXT:-crt}
do
expdate=$($sslcmd x509 -in $file -noout -enddate)
expepoch=$(date -d “${expdate##*=}” +%s)
certificatename=${file##*/}
getExpiry $expepoch ${certificatename%.*}
done
elif [[ $CONFIG -eq 1 ]]; then
#statements
while read line
do
if echo “$line” | \
egrep -q ‘^[a-zA-Z0-9.]+:[0-9]+|^[a-zA-Z0-9]+_.*:[0-9]+’;
then
expdate=$(echo | \
openssl s_client -connect $line 2>/dev/null | \
openssl x509 -noout -enddate 2>/dev/null);
if [[ $expdate = ” ]]; then
#statements
warn “[error:0906D06C] Cannot fetch certificates for $line”
else
expepoch=$(date -d “${expdate##*=}” +%s);
certificatename=${line%:*};
getExpiry $expepoch ${certificatename};
fi
else
warn “[format error] $line is not in required format!”
fi
done < $CONFIGFILE
fi
}
# your script goes here
while getopts “:c:d:w:e:h” options
do
case $options in
c )
CONFIG=1
CONFIGFILE=”$OPTARG”
if [[ ! -e $CONFIGFILE ]] || [[ ! -s $CONFIGFILE ]]; then
#statements
error “$CONFIGFILE does not exist or empty!”
fi
;;
e )
EXT=”$OPTARG”
case $EXT in
crt|pem|cert )
info “Extention check complete.”
;;
* )
error “invalid certificate extention $EXT!”
;;
esac
;;
d )
DIR=1
TARGETDIR=”$OPTARG”
[ $TARGETDIR = ” ] && error “$TARGETDIR empty variable!”
;;
w )
WRITEFILE=1
OUTFILE=”$OPTARG”
;;
h )
usage
;;
\? )
usage
;;
: )
fatal “Argument required !!! see \’-h\’ for help”
;;
esac
done
shift $(($OPTIND – 1))
#
calcEndDate
#finally print the list
if [[ $WRITEFILE -eq 0 ]]; then
#statements
printExpiry ${expcerts[@]}
else
printExpiry ${expcerts[@]} > $OUTFILE
fi
#####
O script irá auxiliar a muitos administradores de segurança gerencie a lista de certificados ssl a serem renovados.
Espero ter contribuído com este artigo a facilitar a vidas dos administradores de TI.
Adriano Valério L. Frare – Pki consultant / BlockChain / Security / Pen Test
Fonte: https://opensource.com
[button link=”https://cryptoid.com.br/category/ssl-tls/” icon=”fa-lock” side=”left” target=”” color=”1abfff” textcolor=”ffffff”]Acesse o maior conteúdo sobre certificados SSL e TLS do Brasil aqui!![/button]